【LeetCode】缀点成线
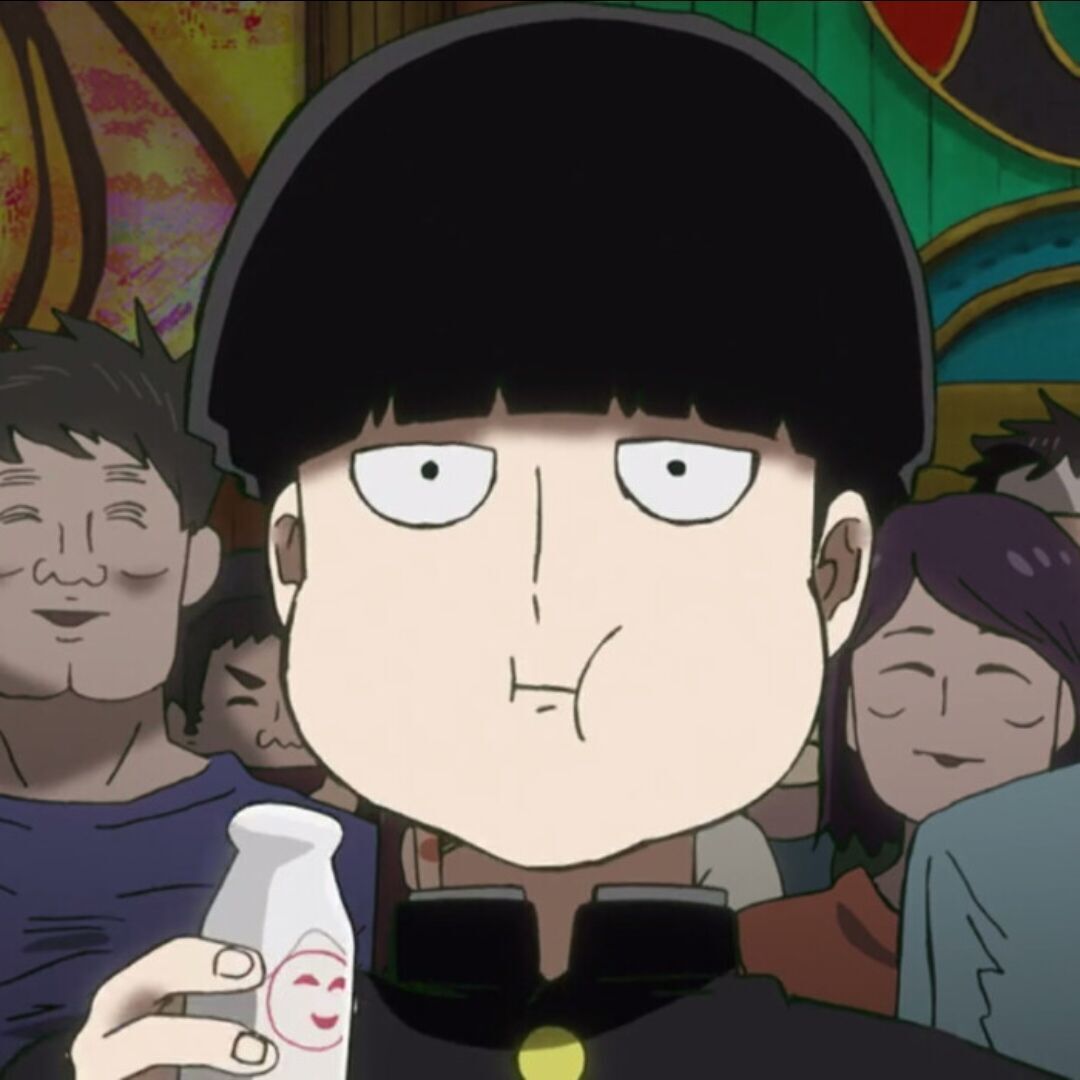
题目描述
给定一个数组 coordinates ,其中 coordinates[i] = [x, y] , [x, y] 表示横坐标为 x、纵坐标为 y 的点。请你来判断,这些点是否在该坐标系中属于同一条直线上。
实现思路一
- 如果每两个点的斜率相同,那么就能确定属于同一条直线上
- 从前两个点开始依次计算斜率并存入数组
- 所有斜率存入数组后,判断数组中的斜率是否相等,如果全部相等则属于同一条直线
具体代码
1 | var checkStraightLine = function(coordinates) { |
实现思路二
- 通过前两个点算出斜率后,再根据直线方程y = ax + b取得截距b
- 代入后续的其他点判断是否满足y = ax + b
具体代码
1 | var checkStraightLine = function(coordinates) { |
- Title: 【LeetCode】缀点成线
- Author: 九號
- Created at : 2024-10-28 14:00:00
- Updated at : 2024-10-30 21:51:48
- Link: https://jhao.me/posts/check-if-it-is-a-straight-line/
- License: This work is licensed under CC BY-NC-SA 4.0.
Comments